Sat Jul 15 2023
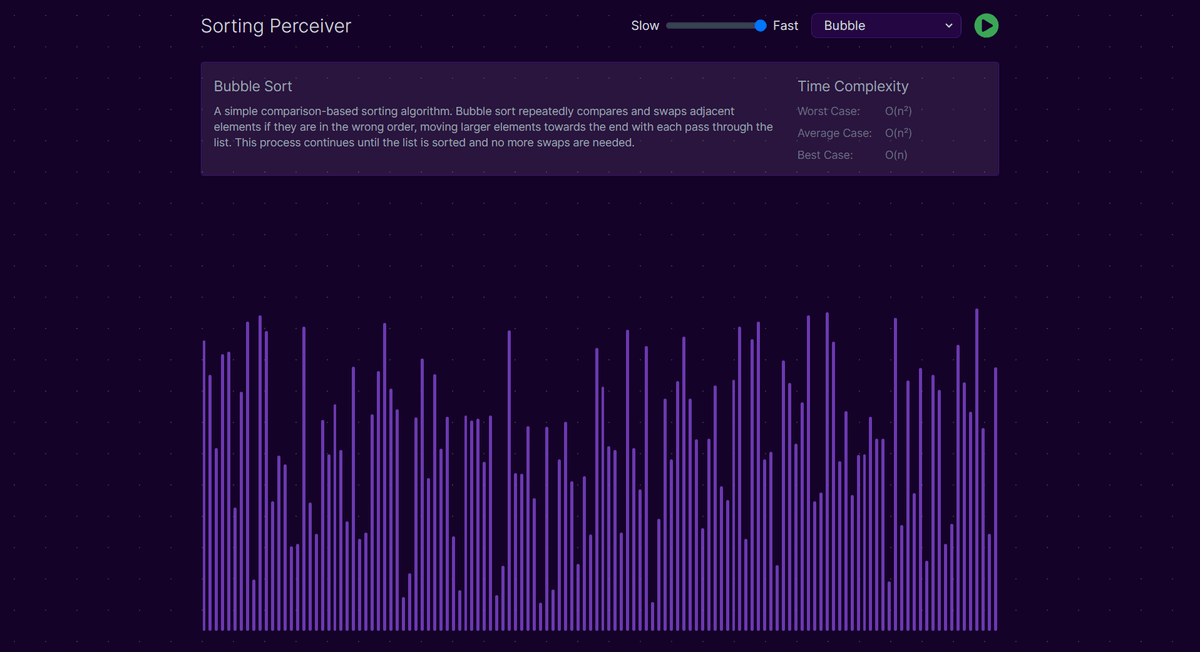
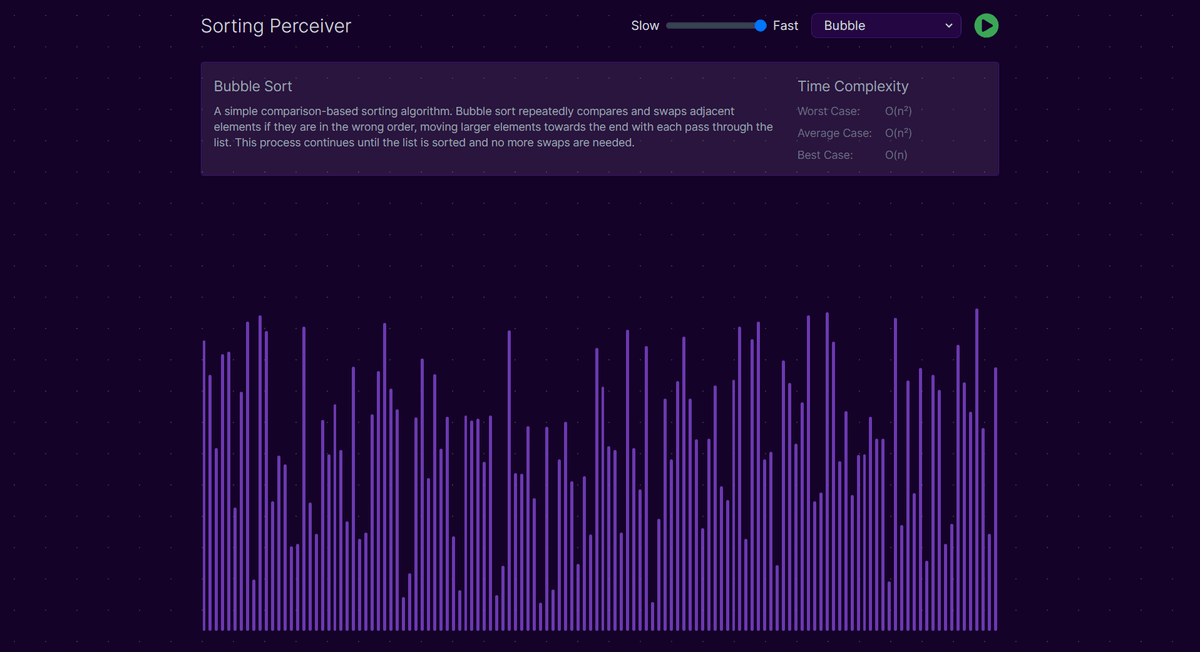
Sorting Perceiver
Next.JS
JavaScript
TypeScript
Tailwind CSS
SCSS
Sorting Perceiver is a web application built using Next.js, designed to illustrate and compare the functionalities of various sorting algorithms. The project serves as an educational tool to help users understand how different sorting techniques work and their efficiencies.
Features
-
Interactive Visualization: View step-by-step visualizations of how each sorting algorithm processes the list.
-
Performance Metrics: Compare the performance of different algorithms on various data sets.
-
Custom Input: Users can input their own data sets to see how the algorithms handle specific cases.
-
Educational Content: Detailed explanations and pseudocode for each algorithm to aid learning.
-
Responsive Design: The application is optimized for desktop and mobile devices, ensuring a seamless user experience across platforms.
Sorting Algorithms Included
-
Bubble Sort: A simple comparison-based algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
-
Selection Sort: An in-place comparison sorting algorithm that divides the input list into two parts: a sorted sublist of items that is built up from left to right at the front (left) of the list, and a sublist of the remaining unsorted items that occupy the rest of the list.
-
Quick Sort: A highly efficient sorting algorithm and is based on partitioning of array of data into smaller arrays. A large array is partitioned into two arrays, one of which holds values smaller than the specified value, say pivot, based on which the partition is made and another array holds values greater than the pivot value.
-
Insertion Sort: A simple sorting algorithm that builds the final sorted array (or list) one item at a time. It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort.
-
Merge Sort: An efficient, stable, comparison-based, divide and conquer sorting algorithm. Most implementations produce a stable sort, meaning that the order of equal elements is the same in the input and output.
Technologies Used
-
Next.js: The project is bootstrapped with create-next-app, leveraging Next.js for its server-side rendering and static site generation capabilities.
-
React: For building interactive user interfaces.
-
CSS/Styled-Components: To style the application.
-
TypeScript: For type-checking and improved code quality.
-
SCSS: For styling and theming the application.
How to Use
-
Clone the repository: git clone https://github.com/abhishekmanhar/Sorting-Perceiver.git
-
Install dependencies: npm install or yarn install
-
Run the development server: npm run dev or yarn dev
-
Open http://localhost:3000: Navigate to the application in your browser.